Use guard statements over else-if
You surely know this, you write a function and at the end you have several blocks IF-ELSE.
Sure, it could be that your programming style is a bit dirty, but maybe you are just working with a language that doesn't support guard like Typescript/Javascript.
Here is an example of what kind of code I mean:
function getClassNameByNumber(number: number): string { if (number > 100) { return "dark"; } else if (number > 50) { return "medium"; } else { return "light"; }}
Fallthrough switch#
Of course, you can probably also use a switch for many of these functions:
function getClassNameByNumber(number: number): string { switch (true) { case number > 100: return "dark"; case number > 50: return "medium"; default: return "light"; }}
guard-style in javascript#
But if you use some kind of guard-style code, it is much easier to read:
function getClassNameByNumber(number: number): string { if (number > 100) return "dark"; if (number > 50) return "medium"; return "light";}
The real guard statement in swift#
Attention, guard is a kind of negative IF. Therefore the logics are reversed here. Only if the guard-statment is True, the function continues.
func getClassName(for number: Int) -> String{ guard number < 100 else { return "dark" } guard number < 50 else { return "medium" }
return "light"}
Conclusion#
I think that this kind of queries are much more readable. Of course you should keep functions as small as possible and in the last return statement you should always keep the most important data based of the return type.
Especially in Swift, I don't want to give away the guard statement anymore.
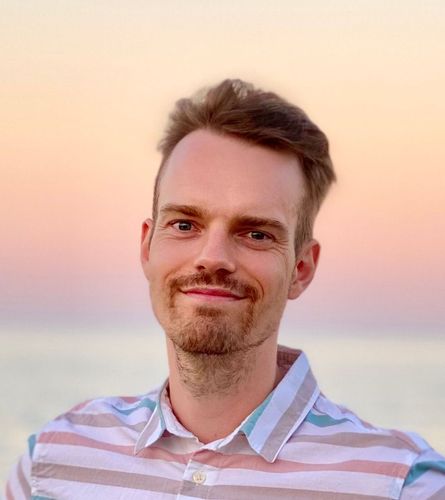